break statement in C is mostly used in a switch statement to terminate the cases present in switch statement. It terminates the loop and transfers the execution process immediately to statement following the loop.
The use of break statement in nested loops terminates the inner loop and the control is transferred to the outer loop. If the break statement is used in nested loops (i.e., loop within another loop), the break statement will end the execution of the inner loop and Program control goes back to the outer loop.
break statement Flowchart:
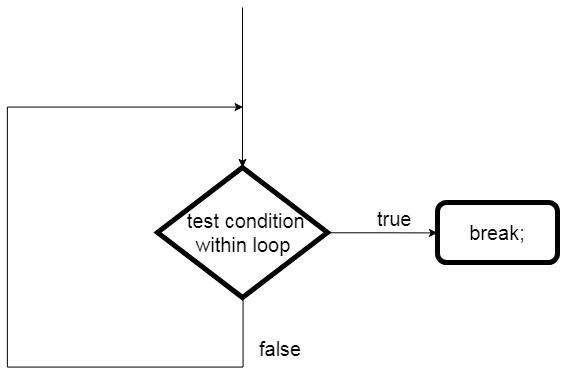
Syntax of break statement:
1 | break; |
Example of break statement in C Program
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | #include <stdio.h> void main() { int i = 1; while (i < 10) { printf("Value of i before break: %d\n", i); if (i == 8) { printf("Loop terminated.\n", i); break; } i++; } } |
The output of break statement in C Programming.
1 2 3 4 5 6 7 8 9 | Value of i before break: 1 Value of i before break: 2 Value of i before break: 3 Value of i before break: 4 Value of i before break: 5 Value of i before break: 6 Value of i before break: 7 Value of i before break: 8 Loop terminated. |