In this tutorial, we will write a program for Magic Number in C. Before that you may go through the following topics in C.
A number is said to be a Magic Number if the sum of the digits of that number, when multiplied by the reverse number of the sum of its digits, is equal to the original number.
Example: 1729 is a magic number.
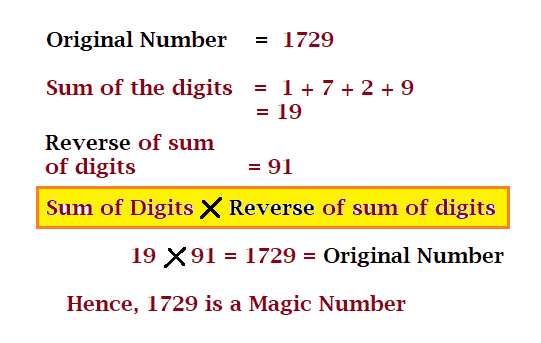
C Magic Number:
In the following C Program to check for magic Numbers, first, take the user input for the number which needed to be checked. Then, we find the sum of the digits of that number and then find the reverse of that sum of the digits.
Lastly, check if the multiplication of reverse and sum of the digits is equal to the original entered number or not. If it is equal then print “Magic Number” else print “Not a magic Number” using the if-else statement in c.
C Program for Magic Number
Question: C program to check whether a number is Magic Number or not
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 | #include <stdio.h> #include <stdlib.h> int main() { int n, temp; int revnum = 0, sumOfTheDigits = 0; //User Input printf("Enter a Number that you want to check:"); scanf("%d", &n); temp = n; //sum of the digits of n number calculation while (temp > 0) { sumOfTheDigits += temp % 10; temp = temp / 10; } //reversing the Sum of the Digits of n temp = sumOfTheDigits; while (temp > 0) { revnum = revnum *10 + temp % 10; temp = temp / 10; } //check for Magic Number and Display if (revnum *sumOfTheDigits == n) printf("%d is a Magic Number.", n); else printf("%d is not a Magic Number.", n); return 0; } |
The Output of magic Number in C Programming.
