Stack Program in C:
This article covers stack implementation using an array in c programming. Here, we will see how to insert in the stack, remove and display the elements in the stack.
The basic operation of stack are:
- PUSH(): This function is used to insert an element on top of the stack.
- POP(): This function is used to remove the element from the top of the stack.
- DISPLAY(): This function is used to display all the elements present in the stack.
Some of the terms related to stack:
- OVERFLOW: It is a state in STACK when the Stack is FULL.
- UNDERFLOW: It is a state in STACK when the Stack is EMPTY.
Lets us understand through C Program for stack using Array.
C Program to Implement Stack using Array with Output
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 | #include <stdio.h> int stack[50], stackSize, topElmt; void push(void); void pop(void); void display(void); int main() { int choices; topElmt = -1; printf("Enter the size for STACK:"); scanf("%d", &stackSize); printf("--------------------------------"); printf("\n STACK OPERATIONS USING ARRAY"); printf("\n--------------------------------"); printf("\nPress 1 to PUSH"); printf("\nPress 2 to POP"); printf("\nPress 3 to DISPLAY"); printf("\nPress 4 to EXIT"); do { printf("\nEnter Your Choice:"); scanf("%d", &choices); switch (choices) { case 1: { //calling PUSH() Function push(); break; } case 2: { //calling POP() Function pop(); break; } case 3: { //calling DISPLAY() Function display(); break; } case 4: { printf("\n---------END----------"); break; } default: { printf("\nWrong Choice."); } } } while (choices != 4); return 0; } //PUSH Function void push() { int x; //Checkfor if STACK is FULL or NOT if (topElmt >= stackSize - 1) { printf("\nSTACK is OVERFLOW"); } else { printf("Enter an Integer that you want to insert: "); scanf("%d", &x); topElmt++; stack[topElmt] = x; } } //POP Function void pop() { //Check if STACK is EMPTY or NOT if (topElmt <= -1) { printf("\nSTACK is UNDERFLOW"); } else { //displaying removed element from top oa a STACK printf("\nThe popped(removed) elements is %d", stack[topElmt]); topElmt--; } } //DISPLAY Function void display() { int i; if (topElmt >= 0) { printf("The Elements present in a STACK are: "); for (i = topElmt; i >= 0; i--) { printf("\n%d", stack[i]); } printf("\nEnter Your Choice"); } else { printf("\n The STACK is empty"); } } |
The output of implementing stack using array in C program.
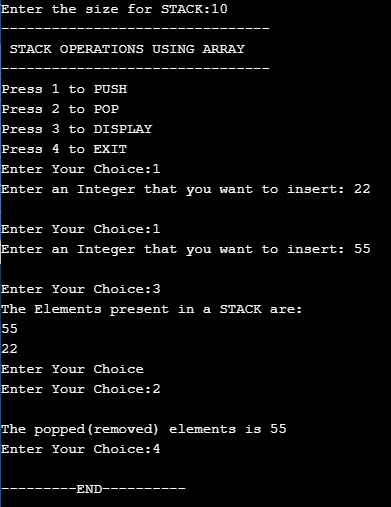