In this tutorial, you will learn about the Bubble Sort and how to implement bubble sort in C Program.
Question:
Write a c program to implement bubble sort.
Sorting is a technique for organizing the elements in an increasing or decreasing order.
Bubble Sort Algorithm:
Bubble Sort is a comparison-based algorithm in which the adjacent elements are compared and swapped to maintain the order or found in the wrong order.
Bubble sort compares the first element with the next one and if found in the wrong order then that compared element in an array are swapped. This algorithm traverse through the entire element in an array.
Time Complexity of Bubble Sort:
- Best case: O(n)
- Average case: O(n^2)
- Worst case: O(n^2)
Bubble Sort in C Program:
Source Code: We will see for descending order. We take the user input for the elements.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 | //Bubble sorting c #include <stdio.h> int main() { int arr[50], numElemt, i, j, swap; printf("Enter maximum number of elements that you want to sort for:\n"); scanf("%d", &numElemt); printf("Enter the Elements:\n"); //elements input for (i = 0; i < numElemt; i++) { scanf("%d", &arr[i]); } //calculation for sorting for decreasing order for (i = 0; i < numElemt - 1; i++) { for (j = 0; j < numElemt - i - 1; j++) { if (arr[j] > arr[j + 1]) { swap = arr[j]; arr[j] = arr[j + 1]; arr[j + 1] = swap; } } } printf("After bubble sort, the sorted elements in decresing order:\n"); //Displaying sorted elements for (i = 0; i < numElemt; i++) printf("%d ", arr[i]); return 0; } |
The output of bubble sorting in c programming.
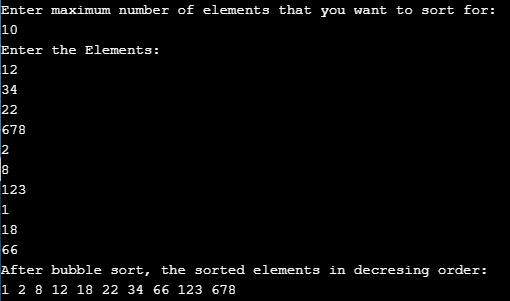