In this tutorial, we will write a program on how to calculate the transpose of a matrix in C program. Let us first understand the transpose of a matrix.
Transpose of a matrix in C:
The new matrix obtained by exchanging the rows and columns of the original matrix is called a transpose matrix. It is a common exercise that every beginner who started to learn C programming must know.
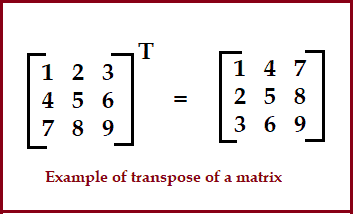
Let us understand through the program in C.
C program to find the transpose of a matrix
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 | #include <stdio.h> int main() { int arr[10][10], transpose[10][10], m, n; printf("Enter number of rows: "); scanf("%d", &m); printf("Enter number of columns: "); scanf("%d",&n); printf("\nEnter matrix elements:\n"); for (int i = 0; i < m; ++i) for (int j = 0; j < n; ++j) scanf("%d", &arr[i][j]); // calculting transpose for (int i = 0; i < m; ++i) for (int j = 0; j < n; ++j) transpose[j][i] = arr[i][j]; // printing the transpose printf("Transpose of entered matrix:\n"); for (int i = 0; i < n; ++i) { for (int j = 0; j < m; ++j) printf("%d ", transpose[i][j]); printf("\n"); } return 0; } |
Output 1: matrix 3×3
Enter number of rows: 3
Enter number of columns: 3
Enter matrix elements:
1 2 3
4 5 6
7 8 9
Transpose of entered matrix:
1 4 7
2 5 8
3 6 9
Output 2: matrix 2×3
Enter number of rows: 2
Enter number of columns: 3
Enter matrix elements:
1 2 3
4 5 6
Transpose of entered matrix:
1 4 2
5 3 6