This is the tutorial to check the number is odd and even in C. A number divisible by 2 is said an even number otherwise the number is called an odd number.
The concept of checking the number using the Modulus operator. First, calculate the number by modulus by 2, and if the Number mod 2 is equal to 0 then it is an even number else not.
Flowchart for odd or even number:
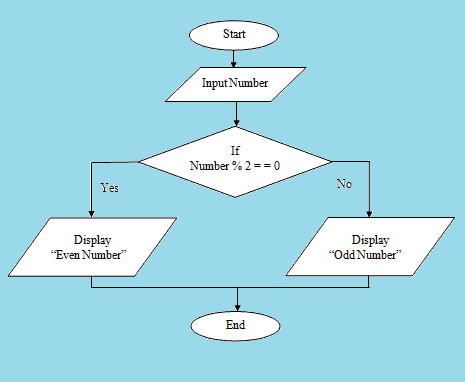
C Program to Check Even or Odd Number
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | #include <stdio.h> int main() { int num; printf("Enter an integer: "); scanf("%d", &num); // condition checking if(num % 2 == 0) printf("%d is an EVEN number", num); else printf("%d is an ODD number", num); return 0; } |
Output:
//Output 1
Enter an integer: 32
32 is an EVEN number
//Output 2
Enter an integer: 11
11 is an ODD number
C Program to Check Odd or Even Using Ternary Operator
The ternary operator works like an if..else statement in C. So the above condition checking of the above program can be done in a single line.
Syntax of the ternary operator. (?:
)
variable num1 = (expression) ? value if true : value if false
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | #include <stdio.h> int main() { int num; printf("Enter an integer: "); scanf("%d", &num); // ternary operator (num % 2 == 0) ? printf("EVEN number") : printf("ODD number"); return 0; } |
Output:
//Output 1
Enter an integer: 52
EVEN number
//Output 2 a
Enter an integer: 7
ODD number