In this tutorial, the program finds out the solutions to the simultaneous equations in two variables. The Linear equations are present in the following form:
- ax+by=c
- px+qy=r
The co-efficient (a, b, c, p, q, r) are taken as an input from the user for the two equations.
C Program to solve Simultaneous Linear Equations in two variables
Source Code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 | #include <stdio.h> int main() { double a, b, c, p, q, r, x, y; //Taking inputs of co-efficient for both equations printf("Enter the coefficents of the first equation of the form ax+by=c\n"); scanf("%lf %lf %lf", &a, &b, &c); printf("Enter the coefficents of the second equation of the form px+qy=r\n"); scanf("%lf %lf %lf", &p, &q, &r); if (((a*q - p *b) != 0) && ((b*p - q*a) != 0)) { //In this case we have a unique solution and display x and y printf("The solution to the equations is unique\n"); x = (c*q - r*b) / (a*q - p*b); y = (c*p - r*a) / (b*p - q*a); //Displaying the value printf("The value of x=%lf\n", x); printf("The value of y=%lf\n", y); } else if (((a*q - p*b) == 0) && ((b*p - q*a) == 0) && ((c*q - r*b) == 0) && ((c*p - r*a) == 0)) { printf("Infinitely many solutions are possible\n"); printf("The value of x can be varied and y can be calculated according to x's value using relation\n"); printf("y=%lf+(%lf)x", (c/b), (-1*a/b)); } else //No possible solution. if (((a*q - p*b) == 0) && ((b*p - q*a) == 0) && ((c*q - r*b) != 0) && ((c*p - r *a) != 0)) printf("No Possible solution\n"); getch(); } |
Let us see two outputs with two different inputs of two equation:
Output1:
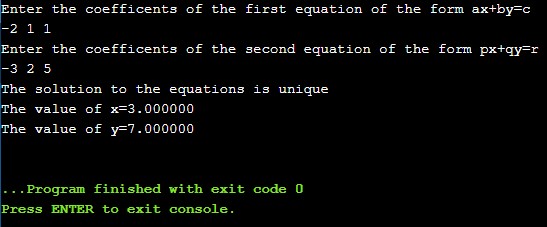
Output2:
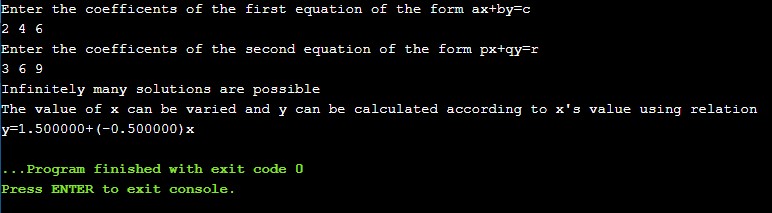