This is a file tutorial where we will write a C program to create a file and write into it. Before that, you may go through the following C topics.
Explanation: The program is based on the file system in C. The program opens the file system in writing mode, hence the “w”. If the file is not present with the name provided then the program will create a file on the same directory.
The program will check if the file is Null or not. If not then, it will ask the user to enter data which later will be inserted into the file. Let us go through the program.
Program to write into a file in C
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | #include <stdio.h> #include <conio.h> void main() { FILE * fptr; char data[100]; fptr = fopen("test.txt", "w"); if (fptr == NULL) { printf("Error occured!"); return; } printf("Enter the data: \n"); fgets(data, 100, stdin); fputs(data, fptr); //writing to a file printf("\nFile created and saved successfully."); fclose(fptr); } |
Output:
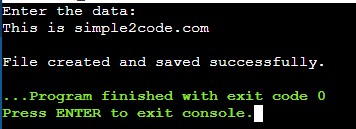
After successful execution of the program, a file named “test.txt” will be created (if not already created) in the same directory where you have stored the source code file. Open that file and you will see the data that you wrote on the program will be present there in the following way.
This is simple2code.com