This is a file tutorial where we will write a program to copy the content from one file to another file in C. Before that, you may go through the following C topics.
Before the program:
Before executing a program you need to create or have a file in the same directory as the source code. If the file from where the program needs to be copied does not exist then the program will show an error message.
So let us create a file with the name “test.txt” and has the following content in it.
Hi! Everyone…
This is simple2code.com
Copy content from one file to another in C program
The program will ask for two file names, one from where the content should be read and to where the content should be written.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 | #include <stdio.h> #include <stdlib.h> int main() { FILE *fptr1, *fptr2; char filename[50], ch; printf("Enter the file name to be read: "); scanf("%s", filename); fptr1 = fopen(filename, "r"); if (fptr1 == NULL) { printf("File does not exists!"); exit(0); } printf("Enter the file name where to be copied: "); scanf("%s", filename); fptr2 = fopen(filename, "w"); if (fptr2 == NULL) { printf("Cannot open file %s \n", filename); exit(0); } ch = fgetc(fptr1); while (ch != EOF) { fputc(ch, fptr2); ch = fgetc(fptr1); } printf("\nContent is copied to %s successfully.", filename); fclose(fptr1); fclose(fptr2); return 0; } |
Output:
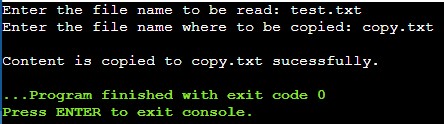
Note: If the file to where the content should be copied is not created before the program then the program will automatically create that file for you.
After successful execution of the program, check the copy.txt file, you will find the same content present in the test.txt file.