Binary search in C programming is to find an element’s position in a sorted Array. The following program for binary search finds the location of a searched element from the array.
Binary search is applied to sorted elements. So if the array is not sorted, you must sort it using a sorting technique. You can use any one of the sorting algorithms in c such as merge or bubble sort etc.
The search for the element that is to be searched and if found in an array, it will print its index number that the key is present.
Binary Search Time Complexity:
- Best case: O(1)
- Average case: O(log n)
- Worst case: O(log n)
C Program for Binary Search
Source Code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 | #include <stdio.h> int main() { int arr[100], i, numElemt, first, last, mid, searchElemt; printf("How many elements do you want?\n"); scanf("%d", &numElemt); printf("Enter the Elements:\n", numElemt); //taking the elements for (i = 0; i < numElemt; i++) scanf("%d", &arr[i]); printf("Enter the specific elements from the list that needed to be searched:\n"); scanf("%d", &searchElemt); first = 0; last = numElemt - 1; mid = (first + last) / 2; while (first <= last) { if (arr[mid] < searchElemt) { first = mid + 1; } else if (arr[mid] == searchElemt) { printf("The elements you are searching is found at location: %d.\n", mid + 1); break; } else { last = mid - 1; } mid = (first + last) / 2; } //if the searching element is not on the list if (first > last) printf("The element you are looking for is not present in the list.\n"); return 0; } |
The output of binary search in c language.
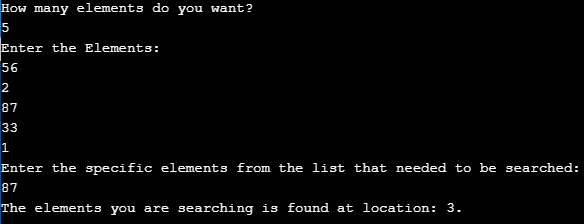