In this article, we will write a C Program for Automorphic Number. The following C programming topic is used in the program below.
A number is said to be an automorphic number if the square of the given number ends with the same digits as the number itself. Example: 25, 76, 376, etc.
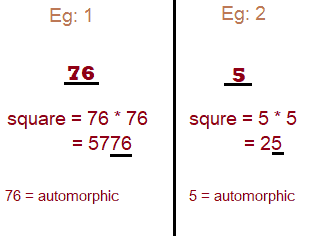
Automorphic Number Program in C
The C program to check automorphic number below takes the number from the user as input and checks accordingly.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 | #include <stdio.h> #include <stdlib.h> #include <math.h> int main() { int num; printf("Enter the number: "); scanf("%d", &num); int sqr, temp, last; int count = 0; sqr = num * num; temp = num; //Counting digits while (temp > 0) { count++; temp = temp / 10; } //calculation int den = floor(pow(10, count)); last = sqr % den; //display if (last == num) printf("%d is an Automorphic number.", num); else printf("%d Not Automorphic number.", num); return 0; } |
Output:
Enter the number: 25
25 is an Automorphic number.