In this tutorial, we will write a C program to count the number of characters words, and lines in a text file. Before that, you may go through the following C topics.
Explanation: The program takes the user input for the file name or file path (if not present in the same directory). Then computes using while loop.
There are three integer variables in a program whose values are incremented by one whenever the condition is fulfilled. When the file reaches the end of the file, the execution control comes out of the loop and finally displays the counted result.
C Program to count Number of Characters Words and Lines in a file
Source code: Write a C program to count characters, words, and lines in a text file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 | #include <stdio.h> #include <stdlib.h> int main() { FILE * file; char fileName[100], ch; int characters, words, lines; printf("Enter the file name: "); scanf("%s", fileName); // open file in read mode file = fopen(fileName, "r"); if (file == NULL) { printf("Unable to open the file"); exit(EXIT_FAILURE); } characters = words = lines = 0; while ((ch = fgetc(file)) != EOF) { characters++; //check for lines if (ch == '\n' || ch == '\0') lines++; //check for words if (ch == ' ' || ch == '\t' || ch == '\n' || ch == '\0') words++; } if (characters > 0) { words++; lines++; } //Display printf("\nTotal Number of characters: %d", characters); printf("\nTotal Number of words: %d", words); printf("\nTotal Number of lines: %d", lines); fclose(file); return 0; } |
Output:
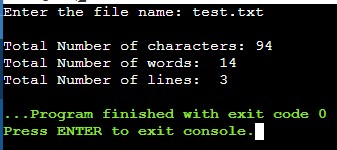
The content inside the test.txt:
Hello!
This is simple2code.com
You can learn coding tutorials and programs from the beginning.