In this tutorial, we will write a java program to calculate the GCD of two numbers.
GCD of two numbers in Java:
GCD(Greatest Common Divisor) or we can say HCF(Highest Common Factor) of two numbers is the largest number(integer) that divides both the number. Or in other words, two numbers that are divisible by the same largest number leaving no remainder.
For example: GCD of 20 and 28 is 4 as shown in the image below.
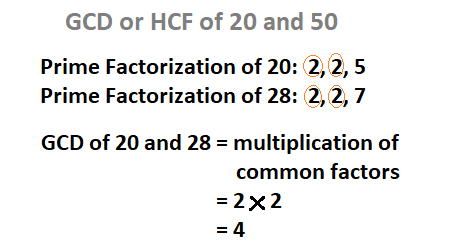
Java program two calculate GCD of two numbers using for loop and if statement
In this example, we take the user input for the two numbers whose GCD needs to be found and store it in num1 and num2. Then iterate through for loop for i less than equal num1 and i less than equal to num2
Inside for loop, we set if statement to check for the divisibility 0f both num1 and num2 by i. If true then we set the value of current i to gcd. This iteration runs until it finds the largest number that divides both num1 and num2.
Program: GCD of Two Number in Java.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | //calculate the gcd of two number import java.util.Scanner; public class GCDJava { public static void main(String[] args) { int num1 , num2, gcd = 1; Scanner sc = new Scanner(System.in); System.out.println("Enter the first number:"); num1 = sc.nextInt(); System.out.println("Enter the second number:"); num2 = sc.nextInt(); for(int i = 1; i <= num1 && i <= num2; ++i) { // Checks if i is factor of both integers if(num1 % i==0 && num2 % i==0) gcd = i; } System.out.printf("G.C.D of %d and %d is %d", num1, num2, gcd); } } |
The Output of GCD of two numbers in java
Enter the first number:
81
Enter the second number:
153
G.C.D of 81 and 153 is 99