The continue statement works like a break statement, instead of terminating the loop the continue statement skips the code in between and passes the execution to the next iteration in the loop.
In case of for
loop, the continue statement causes the program to jump and pass the execution to the condition and update expression by skipping further statement. Whereas in the case of while and do…while loops, the continue statement passes the control to the conditional checking expression.
continue statement Flowchart:
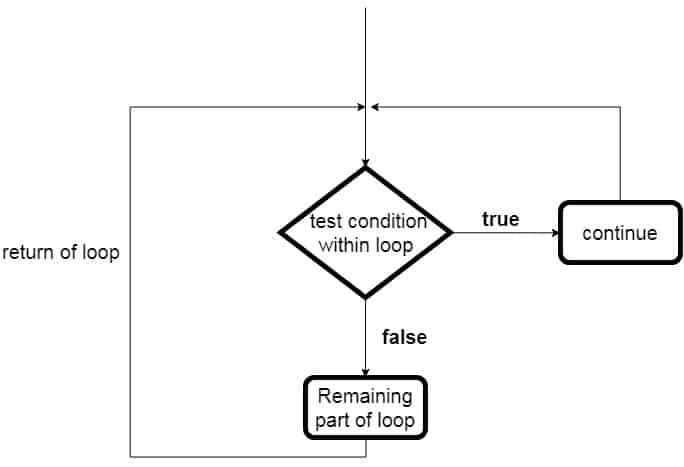
Syntax of continue statement in C++:
1 | continue; |
Example of C++ continue statement
Example: Use of continue in for
loop in C++ programming.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | //C++ program for continue statement #include <iostream> using namespace std; int main() { for (int i = 1; i <= 10; i++) { //continue condition if (i == 6) { //Number 6 is skipped continue; } cout << "Value of i: " << i << endl; } return 0; } |
Output:
1 2 3 4 5 6 7 8 9 | Value of i: 1 Value of i: 2 Value of i: 3 Value of i: 4 Value of i: 5 Value of i: 7 Value of i: 8 Value of i: 9 Value of i: 10 |
As you can see in the above output the 6th iteration is skipped. When the value of i
becomes 6, if statement is executed and hence the continue statement. Then the control is passed to the update expression of the for loop and i
becomes 7.
Example: Use of continue in do-while
loop loop in C++ programming
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | #include <iostream> using namespace std; int main() { int a = 1; // do-while loop do { if (a == 5) { //a incremented and iteration skipped. a += 1; continue; } cout << "value of a: " << a << endl; a += 1; } while (a <= 10); //condition check return 0; } |
Output:
1 2 3 4 5 6 7 8 9 | value of a: 1 value of a: 2 value of a: 3 value of a: 4 value of a: 6 value of a: 7 value of a: 8 value of a: 9 value of a: 10 |
In the above output, 5th iteration is skipped.