A switch statement allows a variable to be tested for equality against multiple values and each of those values is called a case. It can be used instead of nested if...else
..if ladder.
There must be at least one case or multiple cases with unique case values. In the end, it can have a default case which is optional that is executed if no cases are matched.
Switch expression and case value must be of the same type. Each of these case is exited by break keyword which brings the execution out of the switch statement.
The syntax of the switch statement
statement in C#:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | switch (expression) { case value1: //code to be executed; break; //optional case value2: //code to be executed; break; //optional . . . . case valueN: //code to be executed; break; //optional default: code to be executed if all cases are not matched; } |
Switch statement Flowchart:
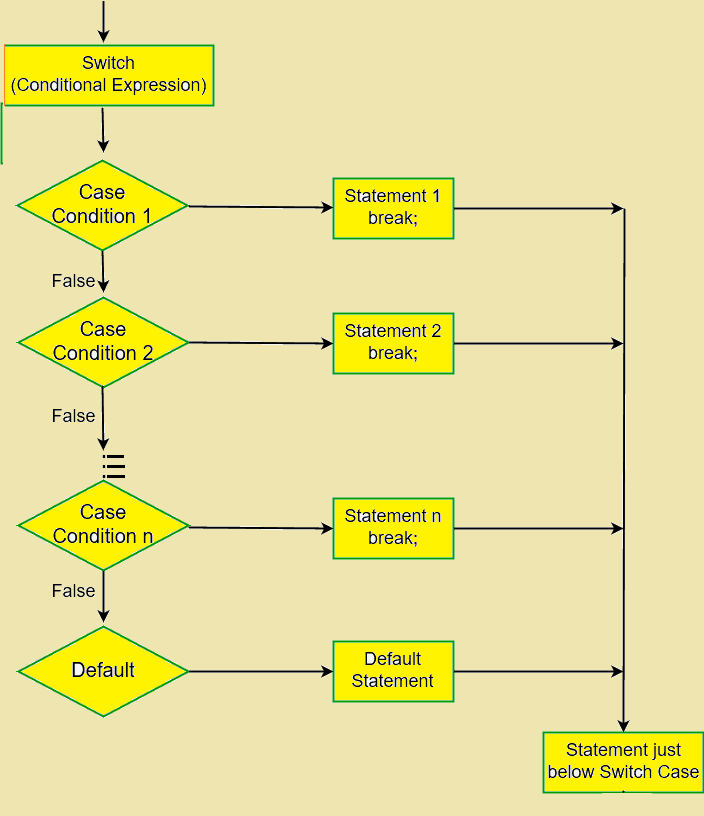
Example of C# switch statement
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 | using System; namespace DecisionStatement { class SwitchStatement { static void Main(string[] args) { char grade_choice = 'D'; switch (grade_choice) { case 'A': Console.WriteLine("Excellent!"); break; case 'B': Console.WriteLine("Very Good!"); break; case 'C': Console.WriteLine("Well done"); break; case 'D': Console.WriteLine("You passed"); break; case 'F': Console.WriteLine("Failed! Better Luck Next time"); break; default: Console.WriteLine("Invalid grade"); break; } } } } |
Output:
1 | You passed |