This statement allows the user to have multiple options to check for different conditions. Here, if one of the if
or else-if
condition is true then that part of the code will be executed and the rest will be skipped. if none of the conditions are true then the final else
statement present at the end will be executed.
The syntax of the if-else-if ladder
statement in C#:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | if(condition1) { //code to be executed if condition1 is true } else if(condition2) { //code to be executed if condition2 is true } else if(condition3) { //code to be executed if condition3 is true } ... else { //final else if all the above condition are false } |
Flowchart diagram for if-else if statement:
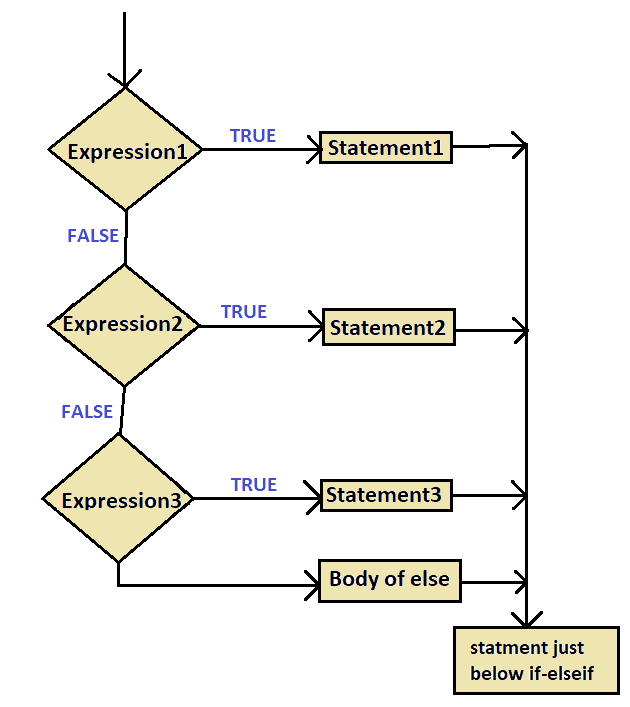
Example of C# if-elseif ladder statement
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | using System; namespace DecisionStatement { class IfElseIfLadder { static void Main(string[] args) { int x = 50; if (x > 70) Console.WriteLine("True, x > 700"); else if (x < 100) Console.WriteLine("True, x < 100"); else if (x == 20) Console.WriteLine("True, x == 20"); else Console.WriteLine("x is not present"); } } } |
Output:
1 | True, x < 100 |