In this tutorial, we will write an ATM program in Java. The program will represent the ATM transaction executed.
Operation available in the ATM Transaction are:
- Withdraw
- Deposit
- Check Balance
- Exit
The user will choose one of the above operations:
- Withdraw is to withdraw the amount from an ATM. The user is asked to enter the amount and after the withdrawal process is complete, we need to remove that amount from the total balance.
- Deposit is to add an amount to the total balance, here also the user enters the amount to be added to the total balance.
- Check Balance means simply display the total balance available in the user’s account.
- Exit is to return the user to the main page from the current transaction mode. For that, we will use
exit(0)
.
ATM program Java
The following program uses the switch statement in java to create a case for each transaction or option.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 | import java.util.Scanner; public class ATMProgram { public static void main(String args[]) { int balance = 5000, withdraw, deposit; Scanner sc = new Scanner(System.in); while (true) { System.out.println("ATM (Automated Teller Machine)"); System.out.println("Choose 1 for Withdraw"); System.out.println("Choose 2 for Deposit"); System.out.println("Choose 3 for Check Balance"); System.out.println("Choose 4 for EXIT"); System.out.print("Choose the operation you want to perform: "); //user choice input int choice = sc.nextInt(); switch (choice) { case 1: System.out.print("Enter the amount to be withdrawn: "); withdraw = sc.nextInt(); //balance need to be with the withdrawn amount if (balance >= withdraw) //for successful transaction { balance = balance - withdraw; System.out.println("Withdrawal successful! Please collect your money."); } else //not enough balance { System.out.println("Insufficient Balance"); } System.out.println(""); break; case 2: System.out.print("Enter amount to be deposited: "); deposit = sc.nextInt(); //adding to the total balance balance = balance + deposit; System.out.println("Your Money has been successfully depsited:"); System.out.println(""); break; case 3: //balance check System.out.println("Available Balance: " + balance); System.out.println(""); break; case 4: //for exit System.out.println("Successfully EXIT."); System.exit(0); } } } } |
Output:
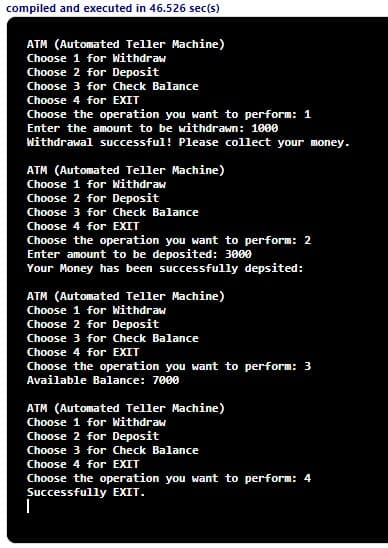