In this tutorial, we will write a Java Program to check Armstrong number. We will write two different programs to check the armstrong number in java.
1. What is an Armstrong number?
2. Java program to check the Armstrong Number(for any digit number) using a while and for.
3. Java program to check the Armstrong Number(using Math.pow() method).
What is an Armstrong Number?
A number is said to be an Armstrong Number if even after the sum of its digits, where each digit is raised to the power of the number of digits is equal to the original number. For example 153, 371, 407, 9474, etc are Armstrong Numbers.
Follow the below calculation.
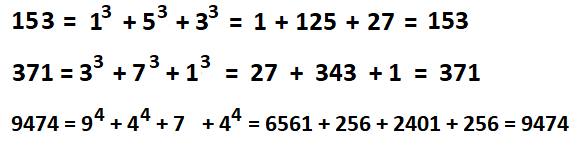
Java program to check the Armstrong Number using while and for loops
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 | import java.util.Scanner; public class ArmstrongNumber { public static void main(String[] args) { int n, num, totalDigits, remainder, result = 0; System.out.println("Enter the Number:"); Scanner scanner = new Scanner(System.in); n = scanner.nextInt(); scanner.close(); //setting the power to the no. of digits totalDigits = String.valueOf(n).length(); num = n; while (num != 0) { remainder = num % 10; int lastDigits = 1; for(int i = 0; i < totalDigits; i++) { lastDigits = lastDigits * remainder; } result = result + lastDigits; num /= 10; } if(result == n) System.out.println(n + " is an Armstrong number."); else System.out.println(n + " is not an Armstrong number."); } } |
Output: You can check for any digit Number.
1 2 3 | Enter the Number: 9474 9474 is an Armstrong number. |
Explanation:
First we create required variables(n, num, totalDigits, remainder, result). Get User Input and store it in n, then we need the number of digits present in that number and store it n totalDigits using code (totalDigits = String.valueOf(n).length();). After that, copy the entered number to num.
Now start the while loop checking num is not equal to zero. While stops as soon as num becomes zero.
After each iteration of the while loop, the last digit of num is stored in remainder for the second use. We initiate an int variable lastDigits = 1.
Now the for loop runs until the totalDigits less than i where is initiated as 0 and increase by 1 after each iteration. Inside for loop lastDigits is multiplied to remainder and the result is added to lastDigits.
After the end of for loop, the value of lastDigits is assigned to the result variable.
and at the end, we divide the num by 10 i.e num /= 10; which will remove the last digit from the number.
This step is continued until the num becomes 0.
At last, we will check with if-else statement whether the result is equal to the original number or not. If it is found equal,l then the entered number is an Armstrong Number.
Java program to check the Armstrong Number using Math.pow() method
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 | //check for armstrong number import java.util.Scanner; public class ArmstrongNumber { public static void main(String[] args) { int n, num, power, remainder, result = 0; System.out.print("Enter the Number: "); Scanner scanner = new Scanner(System.in); n = scanner.nextInt(); scanner.close(); //seting the power to the no. of digits power = String.valueOf(n).length(); num = n; while (num != 0) { remainder = num % 10; result += Math.pow(remainder, power); num /= 10; } if(result == n) System.out.println(n + " is an Armstrong number."); else System.out.println(n + " is not an Armstrong number."); } } |
Output: You can check for any digit Number.
Enter the Number: 370
370 is an Armstrong number
Explanation: In the above program we use the inbuilt method (Math.pow()) which makes it easy for us to calculate the raised power to the number.
The process is the same as explained above, only the difference is instead of for loop we use the method(Math.pow()) as result += Math.pow(remainder, power);
.
If we were to calculate for the only three-digit number then we can simply replace the code:result += Math.pow(remainder, power)
; with result = result + remainder*remainder*remainder;