An Interface in Java is the same as a class, like any other class, an interface can have methods and Variables. But unlike a class, the method in it is an abstract method (only method signature, contain no body), and also by default, the declared variables in an interface are public, static & final.
Uses of Interfaces:
- It is used to achieve abstraction.
- As we have learned that Java does not support multiple inheritance but through the interface, we can achieve multiple inheritance.
- It is also used to achieve loose coupling.
Note: Abstraction cannot be used to create objects. Hence it cannot contain a constructor.
Declaring an Interface in Java:
The keyword ‘interface‘ is used to declare an interface. Syntax of Interface in Java:
1 2 3 4 5 6 | interface interface_name { // declare constant fields, abstract methods } |
Example of interface:
1 2 3 4 5 | interface Dog { public void bark(); // interface method public void run(); // interface method } |
Implementation of Interface in Java:
To implement an interface in a class, we use the keyword ‘implements’ The following example shows the use of implements. In the example below, the class Puppy implements the interface Dog.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | interface Dog { public void bark(); // interface method public void eat(); // interface method } // implementing Dog class Puppy implements Dog { public void bark() { // Body of a bark() method System.out.println("Dog Barks"); } public void eat() { // Body of a eat() method System.out.println("Dog eat Bones"); } } public class MyMainClass { public static void main(String[] args) { Puppy pup = new Puppy(); //Puppy object pup.bark(); pup.eat(); } } |
Output of Interfaces in Java:
Dog Barks
Dog eat Bones
Multiple Interfaces and inheritance in Java:
We know class in java allows only a single extends but with the use of interfaces, we can implement more than one parent interface.
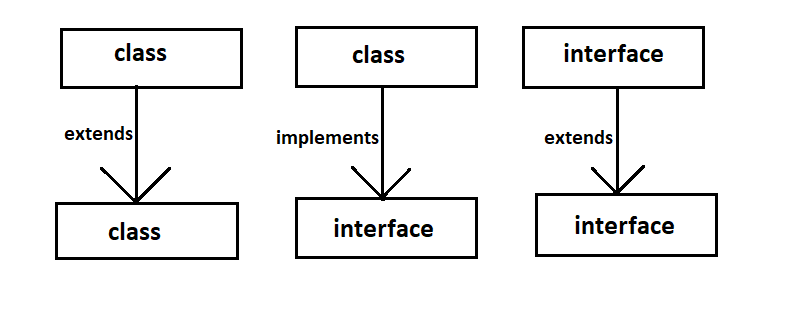
In the diagram above,
- The class extends a class
- The interface extends an interface
- class implements interface
Example of Multiple Interface in Java:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 | interface Interface1 { public void firstMethod(); // interface method } interface Interface2 { public void secondMethod(); // interface method } // InClass "implements" the above two interface class Inclass implements Interface1, Interface2 { public void firstMethod() { System.out.println("Displaying first method"); } public void secondMethod() { System.out.println("Displaying second method"); } } public class MyMainClass { public static void main(String[] args) { Inclass Obj = new Inclass(); Obj.firstMethod(); Obj.secondMethod(); } } |
Output:
Displaying first method
Displaying second method