The applet in Java is a special type of internet-based program, that runs on the web browser and works at the client-side. It is used to make the webpage more dynamic and provide interactive features that cannot be applied by the HTML alone.
- Any applet in Java is a class that extends the java.applet.Applet class.
- An Applet class does not contain the main() method.
- JVM(Java Virtual Machine) is needed to view an applet. To run an applet application, the JVM can use either a plug-in of the Web browser or a separate runtime environment.
Some of the benefits of using Java Applet:
- It is secured. It runs in Sandbox which is a Byte-code verifier.
- Since it works at the client side so less response time.
- It can be executed by the browsers that are running under any platform.
One of the Drawbacks of Java Applet is that for executing applets, plugins are required at the client browser.
Life Cycle of a Java Applet
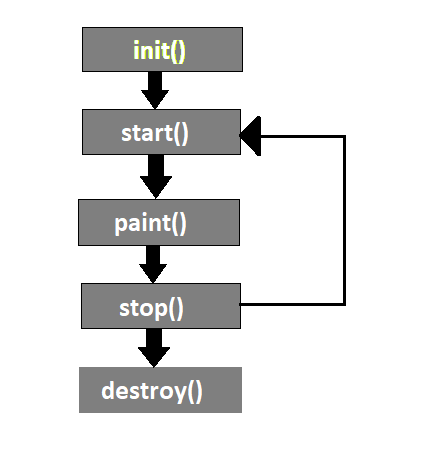
The various stages that an Applet undergoes from its creation to its destruction of objects is known as the Applet life cycle.
Applet life cycle consists of 5 states and each of the states is a signified method and called callback methods.
Following are the states in Applet:
init() method: This method is used for the initialization of an Applet. It is only executed once in a program.
start() method: This method is used to start the Applet and called automatically after the JVM calls init() method. It can be called repeatedly.
paint() method: This method is called immediately after the start(). It is inherited from java.awt, used to paint the object providing the graphic class object.
stop() method: This method is called automatically to stop the Applet. It can also be called repeatedly in the same applet.
destroy() method: This method is used to destroy the Applet and also executed only once in the program.
Applet Class:
All the Applet present is an extension of the java.applet.Applet class. These classes are necessary for the execution of an applet. They include various methods for the applet such as methods to fetch and display images and play an audio clip, resize an applet, to get applet parameters, etc.
Demonstrate a simple Applet example in Java.
1 2 3 4 5 6 7 8 9 | import java.applet.Applet; import java.awt.Graphics; public class FirstApplet extends Applet { public void paint(Graphics g) { g.drawString("First Applet Program", 20, 15); } } |
After saving this code with ‘FirstApplet.java‘. We need to create an HTML file to include this applet. Create an HTML file named first.html within the same folder where you have saved the FirstApplet.java.
1 2 3 4 5 6 7 8 9 10 11 12 | <html> <head> <TITLE> A Simple First Program </TITLE> </head> <body> The output: </body> </html> |
Display Methods in Applet:
As we know applet is displayed in a window and for the input/output operations, they use AWT. To output a string, we use drawString().
The general form for drawString():
1 | void drawString(String message, int x, int y) |
In the above form, this method is written, beginning at x,y which is the location we want the line to begin. This is a graphics class.
- To set a background color of an applet’s window, we use setBackground().
- To set the foreground color, we use setForeground().
The general form of setBackground() and setForeground() are:
1 2 3 | void setBackground(Color newColor) void setForeground(Color newColor) //Here "newColor" denotes the new color. |
The class Color defines the constants shown here that can be used to specify colors:
- Color.black
- Color.blue
- Color.cyan
- Color.darkGray
- Color.gray
- Color.green
- Color.lightGray
- Color.magenta
- Color.orange
- Color.pink
- Color.red
- Color.white
- Color.yellow
The following shows the source code on how to display background color.
1 2 3 | setBackground(Color.blue); setForeground(Color.yellow); |
Note:
The default foreground color is black and the default background color is light gray.